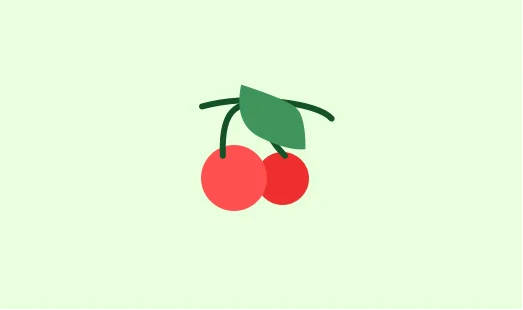
Swift 字符串操作
文章摘要
HeoGPT
这篇文章介绍了 Swift 中常用的字符串操作,包括替换、过滤、去掉空格、分割、拼接和字符串截取。其中,字符串截取使用了 Range 的方法,通过指定起始位置和长度来截取出需要的子字符串。
本文为转载文章,以下内容来源于
swift - 字符串操作(替换、过滤、去掉空格、分割、拼接、字符串截取)
GA_
1 | let s = " / 2 3 4 ? / " |
字符串截取
1 | let timeString = "2016.12.12" |
Range使用举例:
1 | 左到右截取 |
- 感谢你赐予我前进的力量
打赏者名单
因为你们的支持让我意识到写文章的价值🙏
本文是转载或翻译文章,版权归原作者所有。建议访问原文,转载本文请联系原作者。
评论
生成评论
匿名评论
违规举报
✅ 你无需删除空行,直接评论以获取最佳展示效果