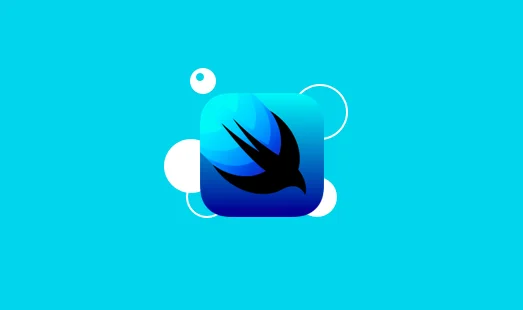
SwiftUI 学习笔记 64:Swift基础复习(四)
文章摘要
HeoGPT
这篇文章介绍了Swift的基础知识复习,包括类的闭包初始化器、反初始化器、循环强引用、弱引用、无主引用、闭包循环引用、尾随闭包、错误枚举及错误捕获、泛型、协议和必要初始化器等内容。
Swift知识基础复习最后一章
类的闭包初始化器{}()
1 | import Cocoa |
反初始化器deinit
被销毁时运行的初始化器
1 | import Cocoa |
循环强引用
想要销毁对象需要断开所有指向他的强引用
1 | import Cocoa |
弱引用weak
想要销毁对象需要断开所有指向他的引用
1 | import Cocoa |
无主引用unowned
1 | import Cocoa |
闭包循环引用 定义捕获列表
1 | import Cocoa |
尾随闭包
1 | import Cocoa |
错误枚举及错误捕获
错误捕捉
1 | import Cocoa |
泛型
可以用一个代码来代替各种类型
1 | func todoit<T>(param: T) -> T { |
协议protocol
类必须要遵守协议
1 | import Cocoa |
必要初始化器required
父类要求子类必须有该初始化器用到required
1 | import Cocoa |
参考资料
- 感谢你赐予我前进的力量
打赏者名单
因为你们的支持让我意识到写文章的价值🙏
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 张洪Heo
评论
生成评论
匿名评论
违规举报
✅ 你无需删除空行,直接评论以获取最佳展示效果