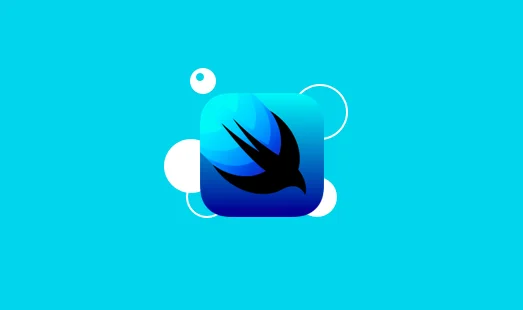
SwiftUI 学习笔记 62:Swift基础复习(二)
文章摘要
HeoGPT
这篇文章介绍了Swift中数组、集合、字典的操作,函数的使用,包括guard拦截语句、inout值传递、函数类型和匿名函数、函数作为返回值和参数、隐式函数及简写等,还涉及到枚举、结构和计算属性等知识点。
补充一下Swift的相关知识。
关于数组的一些操作
1 | import Cocoa |
关于集合的一些操作
1 | import Cocoa |
关于字典的一些操作
1 | import Cocoa |
关于函数的一些操作
1 | import Cocoa |
guard拦截语句
1 | //简单拦截 |
inout 值传递
将函数导入的值变为变量
1 | func double(num: inout Int) { |
函数类型和匿名函数
1 | import Cocoa |
函数作为返回值
1 | import Cocoa |
函数作为参数
1 | func a (num: Int) -> Int{ |
隐式函数及简写
1 | import Cocoa |
关于枚举的一些操作
1 | import Cocoa |
关于结构的一些操作
1 | import Cocoa |
计算属性
1 | import Cocoa |
参考资料
- 感谢你赐予我前进的力量
打赏者名单
因为你们的支持让我意识到写文章的价值🙏
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 张洪Heo
评论
生成评论
匿名评论
违规举报
✅ 你无需删除空行,直接评论以获取最佳展示效果