这篇文章介绍了如何使用SwiftUI创建一个带有进度的圆环,包括设置图形描边、添加线性渐变、旋转和垂直翻转等步骤。文章还展示了如何在整个结构中添加包含其他变量的变量/常量,并添加动态变化部分结束后的代码。
带有进度的圆环实现方式
设置图形描边
1 2
| Circle() .stroke(Color.red, style: StrokeStyle(lineWidth: 5, lineCap: .round, lineJoin: .round, miterLimit: .infinity, dash: [20,0], dashPhase: 0))
|
添加线性渐变
1
| LinearGradient(gradient: Gradient(colors: [Color(#colorLiteral(red: 0.3647058904, green: 0.06666667014, blue: 0.9686274529, alpha: 1)), Color(#colorLiteral(red: 0.8078431487, green: 0.02745098062, blue: 0.3333333433, alpha: 1))]), startPoint: .topLeading, endPoint: .bottomTrailing)
|
添加旋转
1
| .rotationEffect(Angle(degrees: 90))
|
垂直翻转
1
| .rotation3DEffect(Angle(degrees: 180), axis: (x: 1, y: 0, z: 0))
|
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| import SwiftUI
struct RingView: View { var body: some View { ZStack { Circle() .stroke(Color(#colorLiteral(red: 0.7762585282, green: 0.7716453075, blue: 0.7798054218, alpha: 0.3909460616)), style: StrokeStyle(lineWidth: 5)) .frame(width: 44, height: 44) Circle() .trim(from: 0.2, to: 1) .stroke( LinearGradient(gradient: Gradient(colors: [Color(#colorLiteral(red: 0.3647058904, green: 0.06666667014, blue: 0.9686274529, alpha: 1)), Color(#colorLiteral(red: 0.8078431487, green: 0.02745098062, blue: 0.3333333433, alpha: 1))]), startPoint: .topLeading, endPoint: .bottomTrailing), style: StrokeStyle(lineWidth: 5) ) .rotationEffect(Angle(degrees: 90)) .rotation3DEffect(Angle(degrees: 180), axis: (x: 1, y: 0, z: 0)) .frame(width: 44, height: 44) .shadow(color: Color(#colorLiteral(red: 0.2196078449, green: 0.007843137719, blue: 0.8549019694, alpha: 1)).opacity(0.1), radius: 3, x: 0, y: 3) Text("80%") .font(.caption) .fontWeight(.bold) } } }
|
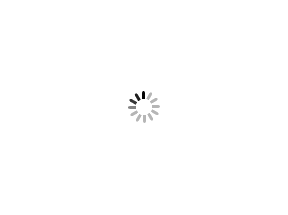
在整个结构中添加包含其他变量的变量/常量
因为需要返回View类型,所以可以在视图前面添加return来在里面添加包含变量的变量/常量
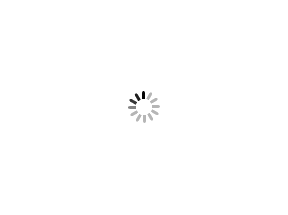
添加动态变化部分结束后代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
|
import SwiftUI
struct RingView: View { var width: CGFloat = 200 var percent: CGFloat = 88 var color1 = #colorLiteral(red: 0.3647058904, green: 0.06666667014, blue: 0.9686274529, alpha: 1) var color2 = #colorLiteral(red: 0.8078431487, green: 0.02745098062, blue: 0.3333333433, alpha: 1) var body: some View { let mutiplier = width / 44 let progress = 1 - percent / 100 return ZStack { Circle() .stroke(Color.black.opacity(0.1), style: StrokeStyle(lineWidth: 5 * mutiplier)) .frame(width: width, height: width) Circle() .trim(from: progress, to: 1) .stroke( LinearGradient(gradient: Gradient(colors: [Color(color1), Color(color2)]), startPoint: .topLeading, endPoint: .bottomTrailing), style: StrokeStyle(lineWidth: 5 * mutiplier, lineCap: .round, lineJoin: .round) ) .rotationEffect(Angle(degrees: 90)) .rotation3DEffect(Angle(degrees: 180), axis: (x: 1, y: 0, z: 0)) .frame(width: width, height: width) .shadow(color: Color(color1).opacity(0.1), radius: 3, x: 0, y: 3) Text("\(Int(percent))%") .font(.system(size: 14 * mutiplier)) .fontWeight(.bold) } } }
struct RingView_Previews: PreviewProvider { static var previews: some View { RingView() } }
|
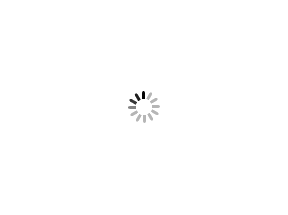
参考:DesignCode