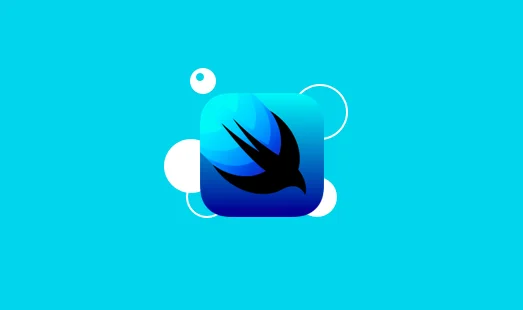
SwiftUI 学习笔记 04:循环
文章摘要
HeoGPT
这篇文章介绍了SwiftUI中的循环,包括for循环、while循环、repeat循环以及如何退出循环或跳过循环项等操作。同时还介绍了如何在嵌套循环中使用标签来退出循环。文章详细讲解了循环的使用方法和注意事项,适合SwiftUI初学者阅读。
罗胖:“直面挑战,躬身入局,皆为我辈中人。”
for循环-普通循环
for循环在Swift中是非常常见的循环。
1 | let count = 1...10 |
你也可以循环数组等。如果你不需要创建类似上面例子的number
数据,可以使用下划线代替,这样swift就不会创建这个多余的数据了,例如:
1 | let albums = ["Red", "1989", "Reputation"] |
while循环-条件循环
while会一直循环到满足条件为止不再循环。
1 | var number = 1 |
repeat循环-至少执行一次的条件循环
有的时候我们需要这个循环至少执行一次,一次以后需要根据条件来判断是否继续运行。我们可以用repeat循环来进行,例如:
1 | var number = 1 |
这个例子可能无法感知它的作用,可以看这个例子:
1 | while false { |
这段代码永远不会运行,因为false始终为false。使用repeat就可以让它运行至少一次:
1 | repeat { |
调用数组的一个repeat循环,例:
1 | var scales = ["A", "B", "C", "D", "E"] |
退出循环
在swift中你可以使用break
来退出循环,例如:
- 这是一个完整的循环
1 | var countDown = 10 |
- 这是一个中断的循环
1 | while countDown >= 0 { |
退出循环中循环(退出嵌套循环)
在swift我们经常嵌套循环,添加标签有助于我们在一定条件时退出这个循环。例如:
- 1.我们创建一个循环
1 | for i in 1...10 { |
- 2.我们在循环外部添加一个标签
1 | outerLoop: for i in 1...10 { |
- 3.通过标签来退出循环
1 | outerLoop: for i in 1...10 { |
跳过该循环项继续循环
在swift中使用continue
来跳过循环,例如:
1 | for i in 1...10 { |
相比break终止了整个循环来说,continue仅仅是让该循环项跳过,继续进行下一次循环。
无限循环
无限循环很常见,我们可以使用while true {}
来进行无限循环,例如:
1 | var counter = 0 |
在273时中断循环。
总结
- 循环使我们可以重复代码,直到条件为假。
- 最常见的循环是for,它将循环中的每个项目分配给一个临时常数。
- 如果你不需要for循环给你的临时常量,请改用下划线,这样Swift可以跳过该工作。
- 有while循环,你可以为其提供一个明确的条件来检查。
- 尽管它们类似于while循环,但repeat循环始终至少在其主体中运行一次。
- 你可以使用退出单个循环break,但是如果你有嵌套循环,则需要使用,break然后再加上在外部循环之前放置的任何标签。
- 你可以使用循环跳过项目continue。
- 直到你要求无限循环,循环才结束,并使用进行循环while true。确保你有条件终止无限循环!
参考资料
- 感谢你赐予我前进的力量
打赏者名单
因为你们的支持让我意识到写文章的价值🙏
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 张洪Heo
评论
生成评论
匿名评论
违规举报
✅ 你无需删除空行,直接评论以获取最佳展示效果