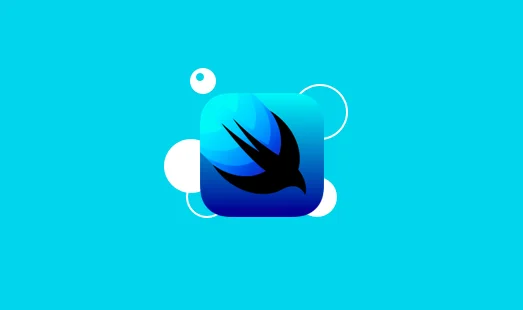
SwiftUI 学习笔记 03:运算符和条件
始终看目标,并不遥远。加油!
算数运算符
在Swift中可以使用算数运算符。
1 | let firstnum = 12 |
当然,也可以多次运算,例如:
1 | let result = 1 + 1 + 1 //结果为3 |
要注意输出的数字为整数(Int)还是浮点数(Double),例如:
1 | let num = 5 * 1 //整数 |
运算符重载
在Swift中运算符的作用可以取决于你给予的值,例如:
1 | let num = 12 + 12 |
你也可以连接字符串,例如:
1 | let firstName = "Hong" |
你可以连接数组,例如:
1 | let peo1 = ["A","B","C"] |
输出值为["A","B","C","D","E","F"]
注意,在Swift中不能多种类型混用。
一些反面例子
let result = false + false //不能对布尔值使用加法
let result = [“North”, “South”] * 2 //不能将字符串数组襄城
复合赋值运算符
在Swift中,可以针对相同变量使用+=
这种运算符进行复合赋值运算,例如:
1 | var num = 100 |
num输出值为90
,成功的缩写了num = num - 10
,这个在很多其他语言几乎也是如此。
你也可以处理字符串,例如:
1 | var txt = "It is sunny " |
txt输出为It is sunny today.
比较运算符
在Swift中有很多用于比较的运算符,例如:
1 | let firnum = 10 |
因为字符串有着字母顺序,所以我们甚至可以来比较字符串大小,例如:
1 | "Taylor" <= "Swift" |
判断语句
我们可以通过比较运算符来添加if
、else if
、else
的条件语句判断,例如:
1 | let firstCard = 11 |
注意:整数(Int)与浮点数(Double)是不能比较的。
“且”和“或”
在数学中我们知道“且”和“或”,同样,在Swift中,也是有“且”和“或”的,分别对应的符号是&&
和||
。
且:两项都为true则为true
或:两项至少又一个为true为true
例如判断两个人的年龄是否都超过固定值:
1 | let age1 = 12 |
判断两个人的年龄是否至少有一个超过固定值:
1 | if age1 > 18 || age2 > 18 { |
三次运算
在Swift中我们甚至可以一口气进行三次运算。例如:
1 | let firstCard = 11 |
?
代表了true,:
代表了false。
所以,上方的代码可以用二次运算的方式进行书写,例如:
1 | if firstCard == secondCard { |
切换语句(多条件处理)
在有非常多条件的时候我们可以使用switch
来进行处理,只需写一个条件就可以处理很多情况。使用default
可以应对你没有列出的可能性。例如:
1 | let phone = "xiaomi" |
输出值为xiaomi is good
,正常情况下switch只会执行这里面的一种情况。如果想要在他处理完之后仍然继续向下处理,那么需要在case最后添加fallthrough
,例如:
1 | let phone = "xiaomi" |
输出值为
xiaomi is good your phone is good
注意:必须提供default的值
范围计算
Swift有两种范围计算的方法,一种是...
一种是..<
。例如:
1...5
代表了1、2、3、4、5;
1..<5
代表了1、2、3、4
例如:
1 | let score = 85 |
总结
- Swift具有用于进行算术和比较的运算符;他们大多像你已经知道的那样工作。
- 算术运算符有多种变体,可以在适当的位置修改其变量:+=,-=等等。
- 你可以使用if,else和else if根据条件的结果运行代码。
- Swift具有三元运算符,该运算符将校验与正确和错误的代码块结合在一起。尽管你可能会在其他代码中看到它,但我不建议你自己使用它。
- 如果你有多个条件使用相同的值,则通常更容易使用switch。
- 你可以使用进行范围调整..<,…具体取决于应排除还是包括最后一个数字。
参考资料
- 感谢你赐予我前进的力量